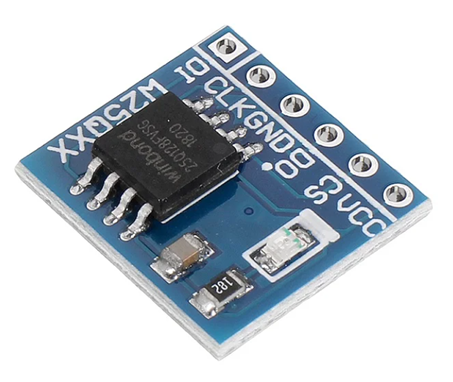
If you are dealing with a flash chip, say from Winbond or Micron, and working with the Arduino IDE, it is very likely that you are using the SerialFlash library developed by PaulStoffregen. Now, you will notice that the library has an eraseAll method and an eraseBlock method. However, there is no method to erase a specific sector. So what do you do if you want to just erase a specific sector?
Turns out that it is actually quite simple. You just need to copy the eraseBlock method from the SerialFlashChip.cpp file and paste it into the same file and change the method name and the instruction code. For W25, according to the datasheet, the instruction code for erasing a sector is 20h as opposed to D8h for erasing a 64 kB block. Thus, the eraseSector function is as follows:
void SerialFlashChip::eraseSector(uint32_t addr)
{
uint8_t f = flags;
if (busy) wait();
SPIPORT.beginTransaction(SPICONFIG);
CSASSERT();
SPIPORT.transfer(0x06); // write enable command
CSRELEASE();
delayMicroseconds(1);
CSASSERT();
if (f & FLAG_32BIT_ADDR) {
SPIPORT.transfer(0x20); //This is 0xD8 in the eraseBlock method
SPIPORT.transfer16(addr >> 16);
SPIPORT.transfer16(addr); }
else {
SPIPORT.transfer16(0x2000 | ((addr >> 16) & 255));
SPIPORT.transfer16(addr); }
CSRELEASE();
SPIPORT.endTransaction();
busy = 2;
}
Of course, don’t forget to add the eraseSector method in the SerialFlashChip class declaration in the SerialFlash.h file.
static void eraseBlock(uint32_t addr);
static void eraseSector(uint32_t addr);
That’s it. You can now erase a only a specific sector. Please note that depending on the chip you are using, the instruction code might differ. Please refer to the datasheet of your flash chip.
For more bite-sized nuggets and detailed tutorials on topics related to IoT, checkout https://iotespresso.com/