You would often feel the need to draw attention to a particular icon on the screen. It would be either for the purpose of highlighting a call to action, or adding more dynamism on the screen. What you are looking for, essentially, is animation. This tutorial will show you how to animate icons such that they expand and shrink periodically. The end result will be something like this:
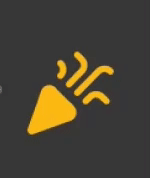
Step 1: Define the Animation controller
First, add the ‘with SingleTickerProviderStateMixin’ to the class declaration. So, the class
_MyClassState extends State<MyClass>
becomes
class _MyClassState extends State<MyClass> with SingleTickerProviderStateMixin
Next, declare the animation controller
late AnimationController animationController;
Finally, assign a value to the animationController in initState().
void initState() {
animationController = AnimationController(
vsync: this,
duration: Duration(milliseconds: 500),
)
..forward()
..repeat(reverse: true);
super.initState();
}
We’ve defined a duration of 500 ms here for the animation. Thus, the motion in each direction, forward and reverse, will take 500 ms.
Step 2: Dispose the animation controller in the dispose() method
void dispose() {
if (mounted) {
try {
animationController.dispose();
} catch (e) {}
}
super.dispose();
}
Step 3: Within build, use the Animation builder to wrap the icon wherever it is being displayed
As you can see from the code below, the size of the icon is a function of the current value of the animation controller. The animation controller’s value goes from 0 to 1 and back again to 0. The icon’s size, therefore, expands from 25 to 30 and then shrinks back to 25.
AnimatedBuilder(
animation: animationController,
builder: (context, child) {
return GestureDetector(
onTap: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => StoryScreen()));
},
child: Icon(
Icons.celebration_rounded,
color: primaryColor,
size: 25 + animationController.value * 5,
),
);
})
The end result, a static celebration icon becomes animated.
Reference: https://stackoverflow.com/a/61338641
If you liked this article, then checkout other articles on https://iotespresso.com.