In order to comply with one or more of the several privacy laws that are coming in, you may have to provide users an option to delete their accounts on your app/website. Once they have signed into your app or website, you may have a button for deleting all the user data. That button, when clicked, can invoke a lambda function that can perform the delete operation for you (provided you are using Cognito for managing user authorization).
In this article, we will see two ways of deleting a user from Cognito using lambda (in the python runtime).
Method 1: Using admin_delete_user
This method can work if your lambda function is associated with a single user pool. The python code will be similar to the one below:
import json
import boto3
client = boto3.client('cognito-idp')
def lambda_handler(event, context):
# TODO implement
user_name = str(event['body']['user_name'])
response = client.admin_delete_user(
UserPoolId='YourUserPoolID',
Username=user_name
)
return response
You need to replace ‘YourUserPoolID’ with the ID of your user pool, and send the user_name in the body, when invoking the lambda. Note that the poolID can be found as soon as you click on the user pool.
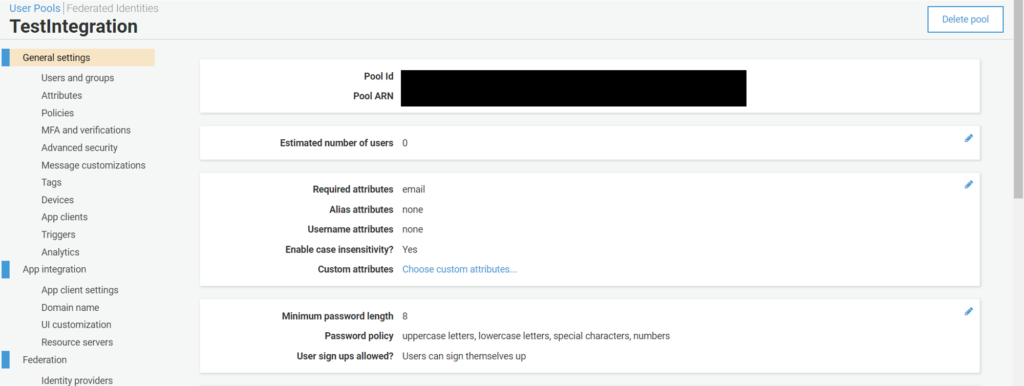
Of course, this lambda will require permission to perform the admin_delete_user operation. You can create a new policy (like the one below) and attach it to the role of this particular lambda.
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "VisualEditor0",
"Effect": "Allow",
"Action": "cognito-idp:AdminDeleteUser",
"Resource": "*"
}
]
}
The advantage (or the drawback, depending on how you see it) of using this method is that it works on any user in the user pool, as long as you have the user name. Therefore, it can have several other use-cases, apart from providing users an option to delete their accounts.
Method 2: Using delete_user
Over here, you require the access token of the user who wants to delete his or her account. When a user logs in to your website or app, Cognito provides an access token unique to that user that can be used to access all the protected resources. The lambda function will look like:
import json
import boto3
client = boto3.client('cognito-idp')
def lambda_handler(event, context):
# TODO implement
access_token = str(event['body']['access_token'])
response = client.delete_user(
AccessToken=access_token
)
return response
Thus, over here, you need to send the access token in the request body. Note that deleting a user by this method means that there is no doubt that the user himself/herself has requested the deletion of their account because the access token is obtained only after a successful sign-in.
Over here, the lambda will need a policy similar to the one below attached to its role, to execute the delete user operation:
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "VisualEditor0",
"Effect": "Allow",
"Action": "cognito-idp:DeleteUser",
"Resource": "*"
}
]
}
This method is less likely to cause accidental deletions of users by the developers because the user sign-in credentials are not known to the developers. At the same time, it cannot be used for force deletions of users (for various reasons, like guideline violations, etc.)
Typically, you would use the delete_user method to provide provisions to users to delete their accounts within the website/ app, and the admin_delete_user method for any deletions to be performed forcefully.
I hope you liked this article. For more tutorials on AWS, check out https://iotespresso.com/category/aws/. Also, if you are planning to become a certified AWS Solutions Architect, I’d recommend that you check out this course on Udemy. I took this course and found the lectures to be lucid, to-the-point, and fun. I hope they will help you as well.